pyboard 的快速参考¶
以下引脚分配适用于 PYBv1.1。您还可以查看其他版本的 pyboard 的引脚排列: PYBv1.0 或PYBLITEv1.0-AC 或 PYBLITEv1.0.
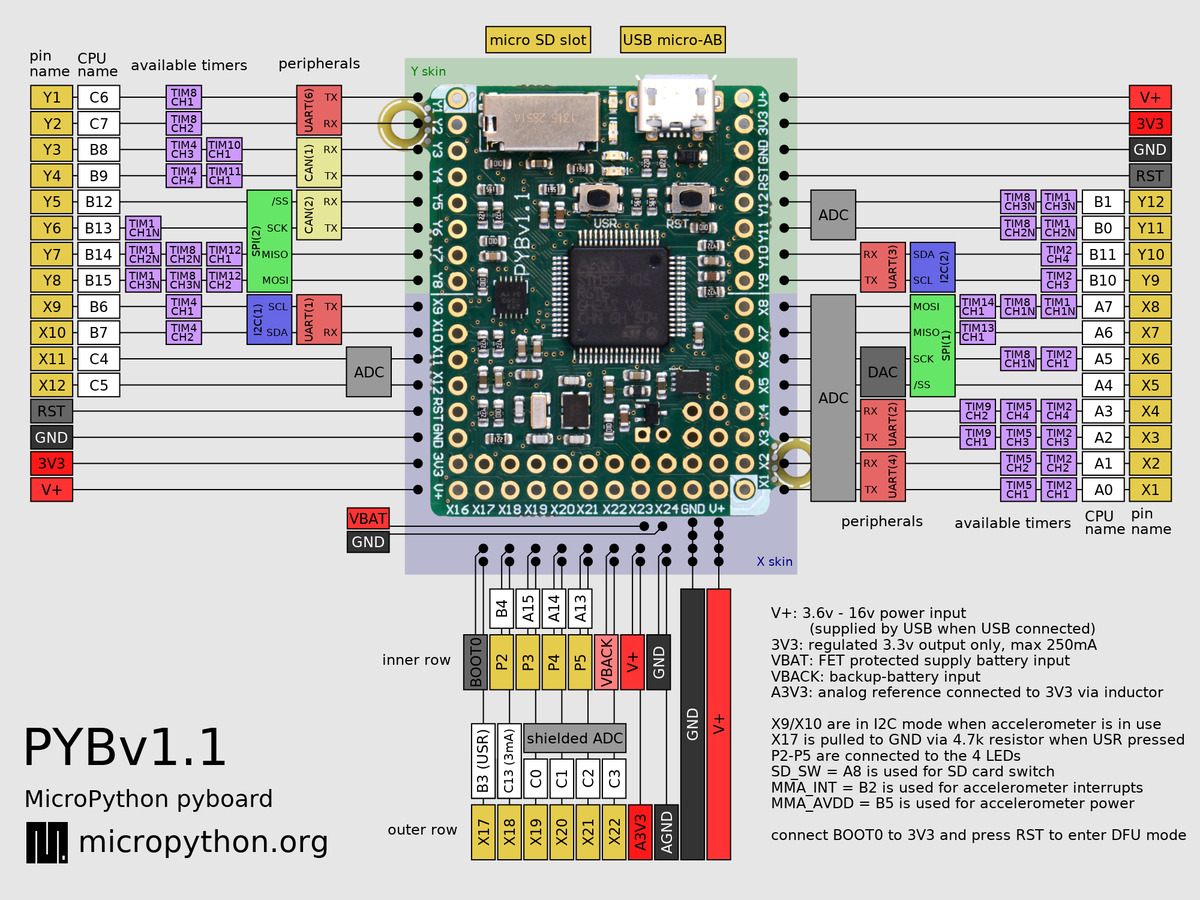
下面是 pyboard 的快速参考。如果这是您第一次使用该板,请考虑先阅读以下部分:
通用板控制¶
见 pyb
.
import pyb
pyb.repl_uart(pyb.UART(1, 9600)) # duplicate REPL on UART(1)
pyb.wfi() # pause CPU, waiting for interrupt
pyb.freq() # get CPU and bus frequencies
pyb.freq(60000000) # set CPU freq to 60MHz
pyb.stop() # stop CPU, waiting for external interrupt
延迟和计时¶
使用 time
模块:
import time
time.sleep(1) # sleep for 1 second
time.sleep_ms(500) # sleep for 500 milliseconds
time.sleep_us(10) # sleep for 10 microseconds
start = time.ticks_ms() # get value of millisecond counter
delta = time.ticks_diff(time.ticks_ms(), start) # compute time difference
内部 LED¶
见 pyb.LED.
from pyb import LED
led = LED(1) # 1=red, 2=green, 3=yellow, 4=blue
led.toggle()
led.on()
led.off()
# LEDs 3 and 4 support PWM intensity (0-255)
LED(4).intensity() # get intensity
LED(4).intensity(128) # set intensity to half
内部开关¶
请参阅 pyb.Switch.
from pyb import Switch
sw = Switch()
sw.value() # returns True or False
sw.callback(lambda: pyb.LED(1).toggle())
引脚和 GPIO¶
请参阅 pyb.Pin.
from pyb import Pin
p_out = Pin('X1', Pin.OUT_PP)
p_out.high()
p_out.low()
p_in = Pin('X2', Pin.IN, Pin.PULL_UP)
p_in.value() # get value, 0 or 1
伺服控制¶
请参阅pyb.Servo.
from pyb import Servo
s1 = Servo(1) # servo on position 1 (X1, VIN, GND)
s1.angle(45) # move to 45 degrees
s1.angle(-60, 1500) # move to -60 degrees in 1500ms
s1.speed(50) # for continuous rotation servos
外部中断¶
请参阅 pyb.ExtInt.
from pyb import Pin, ExtInt
callback = lambda e: print("intr")
ext = ExtInt(Pin('Y1'), ExtInt.IRQ_RISING, Pin.PULL_NONE, callback)
计时器¶
请参阅pyb.Timer.
from pyb import Timer
tim = Timer(1, freq=1000)
tim.counter() # get counter value
tim.freq(0.5) # 0.5 Hz
tim.callback(lambda t: pyb.LED(1).toggle())
RTC(实时时钟)¶
请参阅 pyb.RTC
from pyb import RTC
rtc = RTC()
rtc.datetime((2017, 8, 23, 1, 12, 48, 0, 0)) # set a specific date and time
rtc.datetime() # get date and time
PWM(脉宽调制)¶
from pyb import Pin, Timer
p = Pin('X1') # X1 has TIM2, CH1
tim = Timer(2, freq=1000)
ch = tim.channel(1, Timer.PWM, pin=p)
ch.pulse_width_percent(50)
ADC(模数转换)¶
from pyb import Pin, ADC
adc = ADC(Pin('X19'))
adc.read() # read value, 0-4095
DAC(数模转换)¶
from pyb import Pin, DAC
dac = DAC(Pin('X5'))
dac.write(120) # output between 0 and 255
UART(串行总线)¶
请参阅 pyb.UART.
from pyb import UART
uart = UART(1, 9600)
uart.write('hello')
uart.read(5) # read up to 5 bytes
SPI总线¶
请参阅 pyb.SPI.
from pyb import SPI
spi = SPI(1, SPI.MASTER, baudrate=200000, polarity=1, phase=0)
spi.send('hello')
spi.recv(5) # receive 5 bytes on the bus
spi.send_recv('hello') # send and receive 5 bytes
I2C总线¶
硬件 I2C 可通过I2C('X')
和用于 pyboard 的 X 和 Y 两半 I2C('Y')
。或者,传入外围设备的整数标识符,例如I2C(1)
。通过显式指定
scl
和 sda
引脚而不是总线名称,也可以使用软件 I2C 。有关更多详细信息,请参阅
machine.I2C.
from machine import I2C
i2c = I2C('X', freq=400000) # create hardware I2c object
i2c = I2C(scl='X1', sda='X2', freq=100000) # create software I2C object
i2c.scan() # returns list of slave addresses
i2c.writeto(0x42, 'hello') # write 5 bytes to slave with address 0x42
i2c.readfrom(0x42, 5) # read 5 bytes from slave
i2c.readfrom_mem(0x42, 0x10, 2) # read 2 bytes from slave 0x42, slave memory 0x10
i2c.writeto_mem(0x42, 0x10, 'xy') # write 2 bytes to slave 0x42, slave memory 0x10
注意:对于传统 I2C 支持,请参阅 pyb.I2C。
I2S总线¶
参见 machine.I2S.
from machine import I2S, Pin
i2s = I2S(2, sck=Pin('Y6'), ws=Pin('Y5'), sd=Pin('Y8'), mode=I2S.TX, bits=16, format=I2S.STEREO, rate=44100, ibuf=40000) # create I2S object
i2s.write(buf) # write buffer of audio samples to I2S device
i2s = I2S(1, sck=Pin('X5'), ws=Pin('X6'), sd=Pin('Y4'), mode=I2S.RX, bits=16, format=I2S.MONO, rate=22050, ibuf=40000) # create I2S object
i2s.readinto(buf) # fill buffer with audio samples from I2S device
I2S 类目前作为技术预览版提供。在预览期间,鼓励用户提供反馈。基于此反馈,I2S 类 API 和实现可能会更改。
PYBv1.0/v1.1 有一个 I2S 总线,id=2。PYBD-SFxW 有两条 I2S 总线,id=1 和 id=2。I2S 与 SPI 共享。